Delay element sources loading until they are about to enter the browser display area.
Overview
We say of a resource that is lazy loaded when its loading procedure is deferred until that resource is needed.
Deferring sources loading (for elements that are initially hidden or below the "fold") on a web document can substantially increase the loading time and perceived performance for visitors.
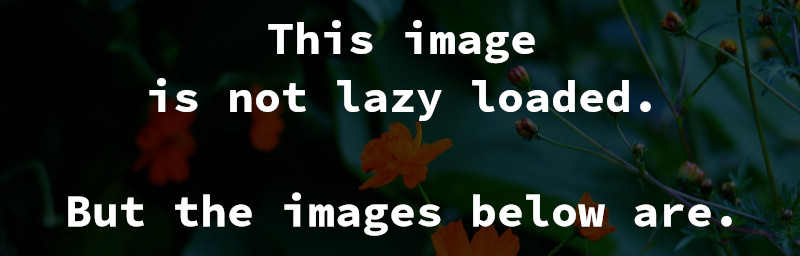
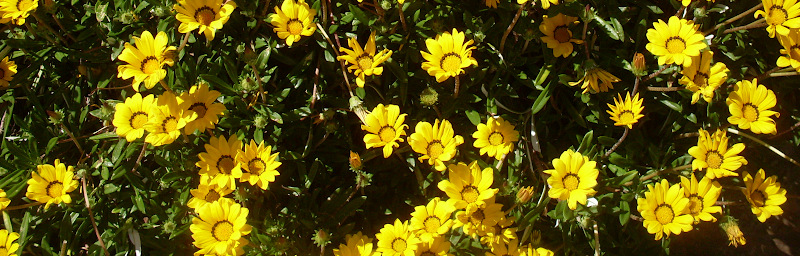
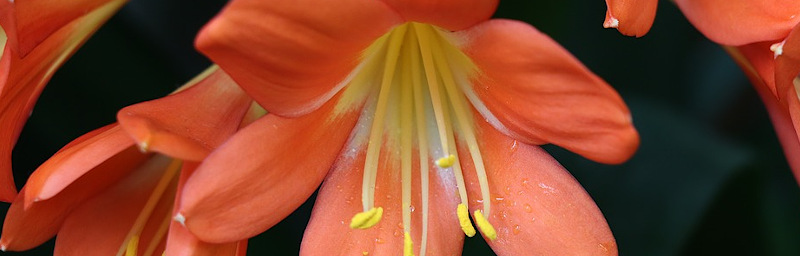
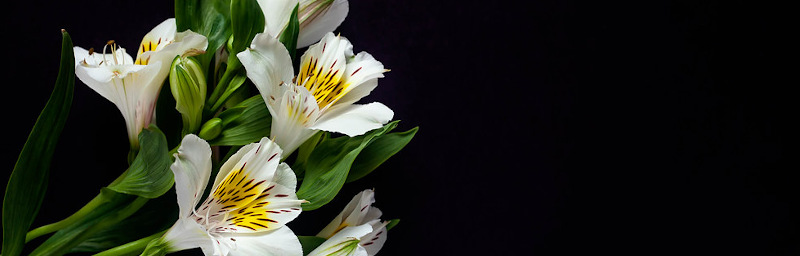
Usage
Dependencies
To get the lazy-loading component working, include the dependencies in your project in the following order:
"design-system": "git+ssh://git@github.com/Selectra-Dev/design-system.git"
import lazyLoading from 'design-system/src/js/00-utilities/lazy-loading.js';
Basic code
When called, this function will check if there are image elements with a
data-src
attribute in the document. This attribute serves two purposes:
- It identifies the element as a lazy loading one.
- It's used to specify the path to the source.
Once the element is about to enter the browser display, its
src
attribute will be replaced and the loading procedure will begin.
<img src="/img/low-quality/placeholder.jpg" data-src="/img/lazy-loading/lazy-1.jpg"/>
img(src='/img/low-quality/placeholder.jpg' data-src='/img/lazy-loading/lazy-1.jpg')
lazyLoading();
In depth
Responsive images
Use the
srcset
and
sizes
attributes to set up lazy
responsive images.
<img data-src="/img/lazy.jpg" data-srcset="/img/lazy_200.jpg 200w, /img/lazy_400.jpg 400w" data-sizes="100w"/>
Lazy picture
Lazy loading picture elements.
<picture>
<source media="(min-width: 1200px)" data-srcset="lazy1200.jpg 1x, lazy2400.jpg 2x">
<source media="(min-width: 800px)" data-srcset="lazy800.jpg 1x, lazy1600.jpg 2x">
<img data-src="lazy.jpg">
</picture>
Lazy video
Lazy loading video elements.
<video controls width="580" data-src="lazy.mp4" poster="lazy.jpg">
<source media="(min-width: 1200px)" data-srcset="lazy1200.jpg 1x, lazy2400.jpg 2x">
<source media="(min-width: 800px)" data-srcset="lazy800.jpg 1x, lazy1600.jpg 2x">
<img data-src="lazy.jpg">
</video>
Lazy iframe
Lazy loading iframe elements.
<iframe data-src="iframe.html" poster="lazy.jpg"><iframe>
HTML attributes
data-src
Use it to specify a replacement value for the
src
attribute of an
img
tag.
<img src="/img/low-quality/placeholder.jpg" data-src="/img/lazy-loading/lazy-1.jpg" />
data-srcset
Use it to specify a
srcset
attribute, either for a
img
or for a
source
tag.
<img data-srcset="/img/lazy-loading/lazy-400.jpg 400w, /img/lazy-loading/lazy-800.jpg 800w" data-sizes="100w" />
data-sizes
Use it to specify a
sizes
attribute, either for a
img
or for a
source
tag.
<img data-srcset="/img/lazy-loading/lazy-400.jpg 400w, /img/lazy-loading/lazy-800.jpg 800w" data-sizes="100w" />