Display content on a modal dialog.
Overview
A modal is a window that is subordinated to the main application window. It's meant to interrupt the workflow on the main application until it's closed.
Did you know?
“OMG” Usage Can Be Traced to 1917.
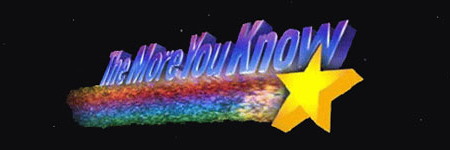
Did you know?
There Was a Successful Tinder Match in Antarctica in 2014.
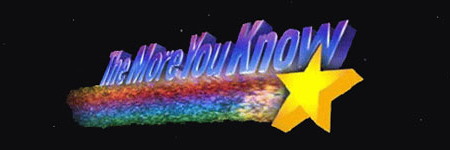
Did you know?
Some Cats Are Actually Allergic to Humans.
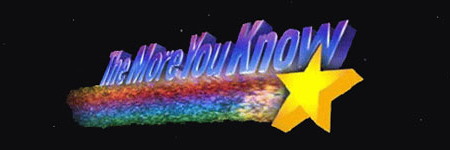
Usage
Dependencies
To get the modal component working, include the dependencies in your project in the following order:
"design-system": "git+ssh://git@github.com/Selectra-Dev/design-system.git"
@import 'design-system/src/scss/03-organisms/modal';
import Modal from 'design-system/src/js/03-organisms/modal';
Basic code
The modal component is composed by two main elements, the trigger and the content holder.
We'll talk about the content holder first.
The content holder represents the dialog window, it will be displayed once the trigger is activated. It's formed by a
div
element with the class
modal__holder
. Inside it we have another
div
with the class
modal__dialog
. Nested inside, there's yet another
div
element, which should have the following attributes:
- The class
modal
, and optionally, one of the size modifier classes. - An unique
Id
. - The
role
aria attribute set todialog
. - The
aria-modal
attribute, set totrue
.
Ok! We have finally set up our modal holder, so... what can we put inside of it?
Let me craft a list for you:
- A cross icon inside a
button
element to close the modal. You can use our button component mixin to create this button, check the documentation for thebtn-close
variety. Thedata-dismiss
attribute for this button must be set tomodal
. - A
div
, with the classmodal__header
. Here you can put ah1-h6
element, with the modal title. - A
div
, with the classmodal__body
, to fill it with the main content. - A
div
, with the classmodal__footer
, for contents related to resolving the modal (going back to the main aplication). Could be a close button, a CTA link...
Nice! Now that we have covered the dialog window, let's talk about the modal trigger.
The trigger is a standard
button
to which you have to set two
data
attributes:
- A
data-toggle
attribute, set tomodal
. - A
data-target
attribute, its value must be set equal to theID
we used for thediv
with the classmodal
.
And that's it! Now all that's left is initializing the component using Javascript.
Instantiate the
Modal
class once, and you are good to go!
<button class="btn btn--md btn--secondary btn--block" data-toggle="modal" data-target="modal-unique-id">Modal trigger</button>
<div class="modal__holder">
<div class="modal__dialog">
<div class="modal modal--sm" id="modal-unique-id" role="dialog" aria-modal="true">
<button class="btn-close btn-close--sm" type="button" aria-label="Close" data-dismiss="modal">
<svg class="icon" aria-hidden="true">
<use xlink:href="/img/sprite.svg#icon-cross"></use>
</svg>
</button>
<div class="modal__header">
<h2 class="modal__title" id="idTitleSmall">Your modal title here.</h2>
</div>
<div class="modal__body">
<p>Your modal content here.</p>
</div>
<div class="modal__footer">
<button class="btn btn--md btn--danger" type="button" data-dismiss="modal">Close me!</button>
</div>
</div>
</div>
</div>
button.btn.btn--md.btn--secondary.btn--block(data-toggle='modal' data-target='modal-unique-id') Modal trigger
.modal__holder
.modal__dialog
.modal.modal--sm#modal-unique-id(role='dialog' aria-modal='true')
+btn-close('sm', 'Close', 'modal')
.modal__header
h2.modal__title#idTitleSmall Your modal title here.
.modal__body
p Your modal content here.
.modal__footer
button.btn.btn--md.btn--danger(type='button' data-dismiss='modal') Close me!
new Modal();
Modifiers
Add the following modifier to change the appearance of a modal.
size
A class modifier for the
modal
class.
We can use it to specify the maximum width of the modal dialog box. If no size modifier is used, the modal dialog will have the width of the document body.
Three sizes are defined:
modal--sm
- Will apply a maximum width of
20rem
.
modal--md
- Will apply a maximum width of
30rem
.
modal--lg
- Will apply a maximum width of
50 rem
.